Unit Testing: A Comprehensive Guide for Software Testing in Software Development
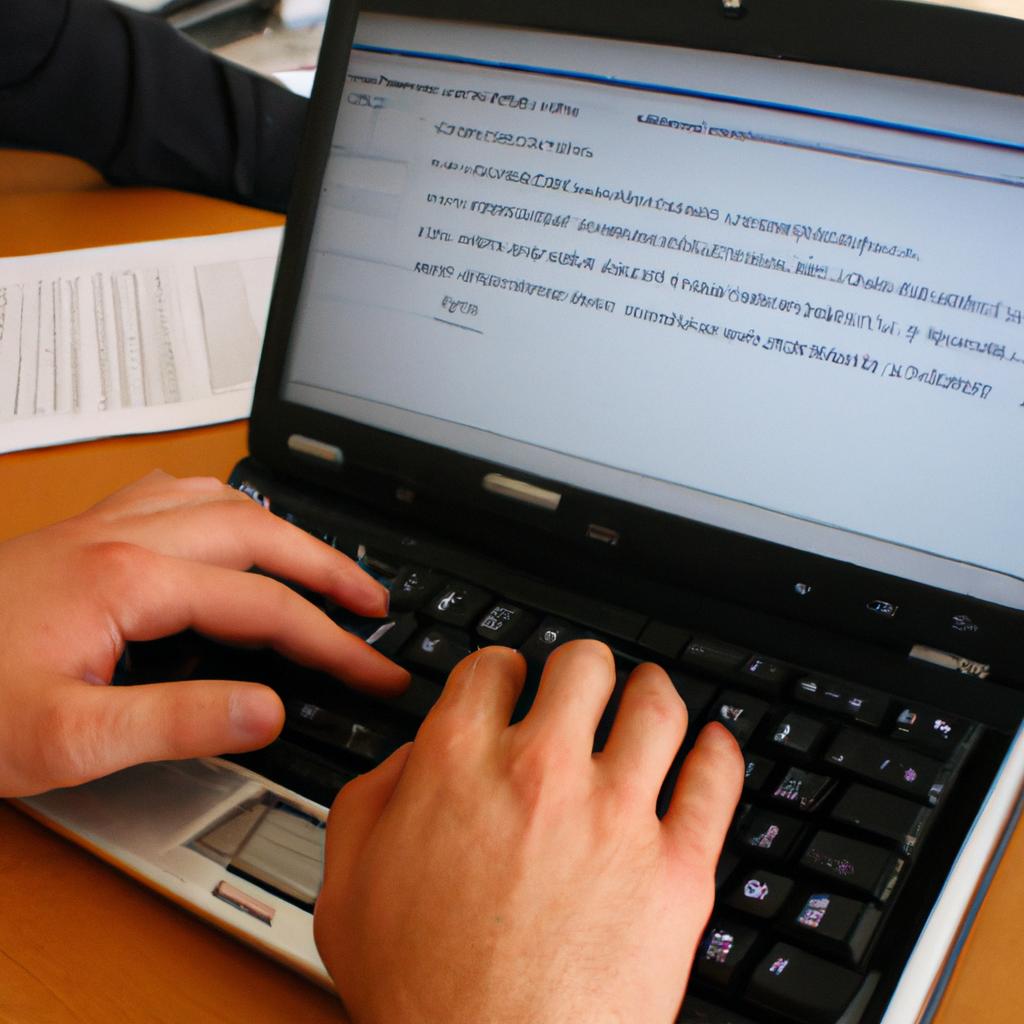
Software testing plays a crucial role in software development, ensuring the quality and reliability of the final product. Amongst various testing techniques, unit testing stands out as an essential practice that focuses on verifying individual units or components of code independently. By isolating these units and subjecting them to rigorous examination, developers can identify defects early in the development process, thus reducing potential risks and enhancing overall software performance.
To illustrate the significance of unit testing, consider a hypothetical case study where a team of developers is working on a complex e-commerce application. The application consists of numerous interconnected modules responsible for different functionalities such as inventory management, order processing, and payment handling. Without proper unit testing, any bugs or errors within these individual modules could go unnoticed until later stages of development or even during production deployment. This lack of early detection not only increases the cost and effort required to fix issues but also poses significant challenges in terms of maintaining system stability and user satisfaction.
Therefore, this comprehensive guide aims to provide software developers with valuable insights into unit testing practices within the context of software development. It will explore various aspects including the benefits of unit testing, common strategies for effective implementation, recommended tools and frameworks available in the industry, best practices for writing test cases, and how to integrate unit testing into the overall software development lifecycle. The guide will also cover topics like test-driven development (TDD), where tests are written before the actual code, and continuous integration (CI) practices that ensure regular and automated execution of unit tests.
Furthermore, this guide will address potential challenges and limitations associated with unit testing, such as handling complex dependencies, dealing with time-dependent or external systems, and managing test coverage. It will provide practical tips for overcoming these challenges and offer guidance on when to use tools like mocking frameworks to simulate external dependencies.
By following the recommendations outlined in this guide, software developers can establish a solid foundation for their unit testing efforts. They will be able to write effective unit tests that validate the correctness of individual code units, improve code maintainability by catching defects early on, enhance collaboration within development teams through shared understanding of code behavior, and ultimately deliver high-quality software products to end-users.
Throughout this guide, I am here to assist you with any questions you may have or provide further clarification on specific topics related to unit testing in software development. Just let me know how I can help!
What is Unit Testing?
Unit testing is a fundamental practice in software development that involves the validation of individual units or components of source code to ensure their correctness and functionality. These units are typically small, self-contained modules or functions within a larger system. By isolating and thoroughly testing each unit, developers can detect defects early on and improve the overall quality of their software.
To illustrate the importance of unit testing, let’s consider an example. Imagine a team developing an e-commerce website where users can browse products, add them to their cart, and proceed to checkout. One critical component is the “add-to-cart” function, which enables users to select items they wish to purchase. Without proper unit testing, there may be undetected issues such as incorrect pricing calculations or failure to update inventory levels when items are added to the cart.
- Ensures functional accuracy: By meticulously examining individual units of code, unit testing helps identify bugs and errors promptly.
- Enhances maintainability: Thoroughly tested units simplify maintenance tasks by reducing complexity and enabling easier debugging.
- Facilitates collaboration: Unit tests serve as documentation for future developers working on the codebase, providing insights into expected behaviors.
- Boosts confidence: Developers gain assurance in their code through successful execution of unit tests, leading to increased trust in the software.
Moreover, it is useful to present information visually using tables. Here is an example table showcasing additional advantages of unit testing:
Advantages | Description |
---|---|
Early bug detection | Identifying issues at an early stage saves time and effort in fixing them. |
Faster development | Resolving problems during coding prevents slowdowns later in the process. |
Improved software design | Writing testable code often leads to better architecture choices. |
Cost-effective | Catching bugs early reduces the expenses associated with fixing them. |
In conclusion, unit testing plays a crucial role in software development by ensuring the correctness and functionality of individual code units. By employing this practice, developers can identify defects early on, enhance maintainability, foster collaboration among team members, and gain confidence in their code.
Next section: Benefits of Unit Testing
Benefits of Unit Testing
Previous section H2:’What is Unit Testing?’
Next section H2:’Benefits of Unit Testing’
Transitioning smoothly from the previous section discussing “What is Unit Testing?” let us now explore the various benefits that unit testing brings to software development. To illustrate these advantages, consider a hypothetical scenario where a team of developers is working on an e-commerce application.
Unit testing provides several significant benefits that contribute to the overall success and quality of software projects:
-
Improved Code Quality: By writing tests at the individual component level, known as units, developers can ensure that each piece of code functions correctly in isolation. This rigorous approach identifies bugs or issues early in the development process, allowing for quick resolution before they become more complex and costly problems.
-
Faster Debugging: With comprehensive unit tests in place, any new changes made during development are immediately validated against existing tests. If a test fails after implementing new functionality, it indicates that something has gone wrong with the recent modifications. These failed tests help pinpoint exactly which part of the code requires debugging or fixing, leading to faster identification and rectification of errors.
-
Facilitates Refactoring: Refactoring involves altering existing code without modifying its external behavior. Without proper test coverage, refactoring can be risky since even small changes may inadvertently introduce defects elsewhere in the system. However, by having well-designed unit tests covering all critical functionalities, developers gain confidence when making structural improvements or optimizing their codebase.
-
Enhanced Collaboration: Unit testing promotes collaboration among team members by establishing clear guidelines and expectations for how different components should interact with one another. Test cases act as living documentation that outlines usage scenarios and expected outcomes, enabling effective communication between developers, testers, and other stakeholders involved throughout the software development lifecycle.
To further emphasize these points visually:
Benefits of Unit Testing |
---|
· Improved code quality |
In conclusion, unit testing offers numerous advantages to software development projects. It enhances code quality by identifying errors early on and facilitating faster debugging when changes are made. Additionally, it enables safe refactoring of existing code while promoting collaboration among team members. With these benefits in mind, let us now delve into the various unit testing frameworks available that can assist developers in achieving effective test coverage.
Unit Testing Frameworks
Transitioning from the previous section, where we explored the benefits of unit testing, let us now delve into the various unit testing frameworks available in software development. To illustrate their importance, consider a hypothetical scenario where a team of developers is working on a complex web application. Without using any unit testing framework, they make changes to different components simultaneously. As a result, when an issue arises, it becomes challenging to identify which specific component caused the problem. Now imagine the same scenario with a well-implemented unit testing framework in place – each component would be tested individually, allowing for easier identification and resolution of issues.
Unit testing frameworks provide essential tools for automating and streamlining the process of running tests on individual units of code. These frameworks offer numerous advantages that greatly enhance the efficiency and effectiveness of unit testing efforts:
- Code reusability: With unit testing frameworks, test cases can be easily reused across multiple projects or modules.
- Test coverage analysis: They enable developers to assess how much of their code has been exercised by tests, ensuring comprehensive coverage.
- Continuous integration support: Unit testing frameworks integrate seamlessly with continuous integration systems like Jenkins or Travis CI, enabling automated execution of tests during build processes.
- Simplified debugging: By isolating errors within small units of code, these frameworks facilitate faster debugging and troubleshooting.
To further highlight the significance of unit testing frameworks in software development, let’s consider some popular options commonly used by developers:
Framework | Language(s) | Key Features |
---|---|---|
JUnit | Java | Annotations-based approach; extensive community support |
NUnit | .NET languages | Attribute-driven model; compatibility with Visual Studio |
Pytest | Python | Concise syntax; supports parallel test execution |
Jasmine | JavaScript | Behavior-driven development (BDD) style; browser compatibility |
In this section, we explored the importance of unit testing frameworks in software development. These frameworks provide valuable tools and features that enhance efficiency and effectiveness during the testing process. In the subsequent section, we will delve into best practices for unit testing, ensuring optimal utilization of these frameworks to achieve high-quality code.
Transitioning into the next section on “Best Practices for Unit Testing,” it is crucial to establish a solid foundation by understanding the significance of proper implementation and execution of unit tests within the context of software development.
Best Practices for Unit Testing
To ensure effective unit testing, it is crucial to follow best practices that promote efficiency and reliability in software development. This section will explore some key strategies that can enhance the quality of unit tests and ultimately contribute to the overall success of a project. By incorporating these best practices, developers can mitigate risks and deliver robust software solutions.
Example Scenario:
Consider a hypothetical scenario where a team of developers is working on an e-commerce application. One critical component of this application is the shopping cart functionality. To verify its correctness, the team decides to implement unit tests using appropriate frameworks. By following best practices, they aim to identify potential bugs early in the development cycle and prevent issues from arising during integration or production stages.
Best Practices:
- Maintain Test Isolation: It is essential to ensure that each unit test remains independent and does not rely on external resources or dependencies. By isolating tests, any failures can be easily pinpointed, making debugging more efficient.
- Use Descriptive Naming Conventions: Clear and descriptive names for your test cases help in understanding their purpose without delving into code details directly.
- Prioritize Code Coverage: Aim for comprehensive coverage by striving to include all possible execution paths in your unit tests. Tools like code coverage analysis can assist in identifying areas with insufficient test coverage.
- Regularly Refactor Tests: As the codebase evolves over time, it’s important to regularly refactor existing tests to align them with current requirements and avoid redundancy.
- Ensures higher confidence in software quality
- Reduces chances of regression bugs
- Facilitates collaboration between team members
- Saves time and effort spent on manual testing
Table – Importance of Best Practices for Unit Testing:
Benefits | Description |
---|---|
Improved Quality | Following best practices ensures thorough testing, leading to enhanced software quality. |
Early Bug Detection | Identifying bugs early in the development process allows for timely resolution and smoother progress. |
Efficient Debugging | Isolated tests make it easier to locate failing components, simplifying the debugging process. |
Enhanced Collaboration | Adhering to best practices fosters better collaboration among team members during testing activities. |
By implementing these best practices, developers can lay a strong foundation for effective unit testing.
Common Mistakes in Unit Testing
Building upon the best practices discussed earlier, it is crucial to be aware of common mistakes that developers often make when implementing unit testing. By understanding these pitfalls and avoiding them, software development teams can ensure the effectiveness and reliability of their unit tests.
One example of a mistake frequently encountered in unit testing involves writing overly complex test cases. In an attempt to cover all possible scenarios, developers may create tests that are difficult to understand or maintain. This complexity not only increases the chances of errors within the test code itself but also hampers future modifications or additions to the system. To avoid this, it is important for developers to focus on simplicity and clarity when designing their unit tests.
To further illustrate potential mistakes, consider the following bullet points:
- Neglecting proper assertions: Failing to include sufficient assertions within test cases can lead to inaccurate results and undermine the purpose of unit testing.
- Ignoring edge cases: Overlooking boundary conditions or exceptional scenarios during test case design can leave critical areas untested.
- Relying solely on production data: Using real data from production environments without considering variations or randomization may result in biased test outcomes.
- Not updating tests alongside code changes: Failing to update existing tests as code evolves can introduce discrepancies between expected and actual behavior.
Mistake | Impact |
---|---|
Writing inadequate documentation for tests | Hampers collaboration and troubleshooting efforts |
Having long-running or slow unit tests | Slows down overall development process |
Creating tightly-coupled or dependent tests | Increases fragility and reduces maintainability |
Neglecting code coverage analysis | Leaves gaps in test coverage |
In summary, by being mindful of common mistakes such as overcomplicating test cases, neglecting proper assertions, ignoring edge cases, and failing to update tests alongside code changes, software development teams can enhance the quality and effectiveness of their unit testing practices. By avoiding these pitfalls, developers can build robust and reliable software systems.
The integration of unit testing in the software development lifecycle will now be explored to understand how it fits into the broader process without disrupting its flow.
Integration of Unit Testing in Software Development Lifecycle
Avoiding the common mistakes mentioned above is crucial for successful unit testing. Once these pitfalls are understood and addressed, it becomes essential to integrate unit testing effectively within the software development lifecycle.
Unit testing plays a vital role in ensuring the quality and reliability of software applications. To achieve this, integrating unit testing into each phase of the software development lifecycle (SDLC) is necessary. Let’s consider an example scenario where a team of developers is working on developing a web-based application that allows users to create and manage their personal blogs.
During the requirements gathering phase, incorporating unit tests can help validate whether the functional requirements align with user expectations. For instance, by writing test cases for blog creation, authors’ credentials verification, or comment moderation functionalities, potential issues can be identified early on. This ensures that any deviations between expected behavior and actual implementation are detected promptly.
- Minimize Risks: By performing thorough unit testing at every stage of SDLC, risks associated with defects and failures can be minimized.
- Enhance Code Quality: Integrating unit tests encourages developers to write cleaner code that adheres to coding standards and best practices.
- Improve Collaboration: When integrated into version control systems or continuous integration tools, unit tests foster collaboration among team members.
- Boost Confidence: Effective integration enables swift detection of bugs and instills confidence in developers as they release more stable versions.
The table below summarizes how integrating unit testing at different stages enhances software development:
Stage | Benefits |
---|---|
Requirements Gathering | Early identification of functionality gaps |
Design | Validation against design specifications |
Implementation | Detection of logical errors and anomalies |
Maintenance | Regression testing for stability assurance |
By integrating unit testing throughout the SDLC, developers can ensure that potential issues are addressed early on and avoid costly bug fixes during later stages. This approach not only enhances code quality but also improves collaboration among team members, ultimately leading to more robust and reliable software applications.
Overall, integrating unit testing within the software development lifecycle is a crucial aspect of ensuring high-quality software products. By doing so, teams can minimize risks, enhance code quality, improve collaboration, and boost their confidence in delivering stable releases.