Design Patterns: Software Development in Object-Oriented Programming
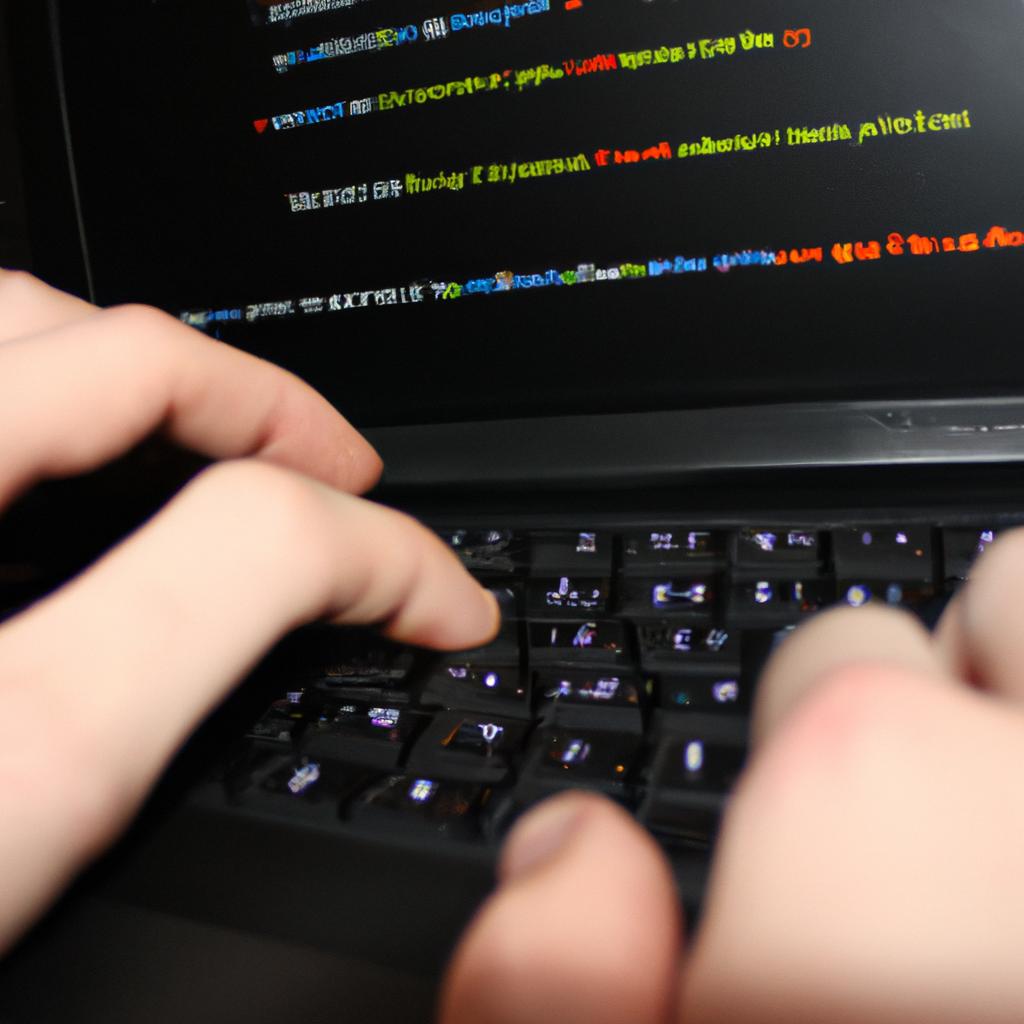
Design patterns play a crucial role in the field of software development, particularly in object-oriented programming. These patterns are reusable solutions to common problems that arise during the design and implementation phases of software development projects. By following established design patterns, developers can enhance their code’s flexibility, maintainability, and reusability. For instance, consider a hypothetical scenario where a team of developers is tasked with building an e-commerce website. Without utilizing any design patterns, they may find themselves repeatedly reinventing certain functionalities such as user authentication or payment processing. However, by applying appropriate design patterns like the Singleton pattern for managing database connections or the Observer pattern for updating product availability notifications, the developers can streamline their development process and create more robust and scalable applications.
In this article, we will delve into the world of design patterns and explore how they contribute to effective software development practices in an object-oriented programming environment. Object-oriented programming (OOP) has become increasingly popular due to its ability to model real-world entities as objects with defined properties and behaviors. However, as OOP projects grow in complexity, it becomes essential to employ well-established design principles and practices to ensure sustainable codebases.
Throughout this article, we will discuss various categories of design patterns commonly employed in OOP projects – such as creational patterns, structural patterns, and behavioral patterns.
Creational patterns focus on the creation of objects and help developers manage object instantiation in a flexible and efficient manner. Examples of creational patterns include the Singleton pattern mentioned earlier, which ensures that only one instance of a class exists throughout the application’s lifecycle. Another example is the Factory pattern, which delegates object creation to subclasses or external components.
Structural patterns deal with organizing classes and objects into larger structures while maintaining their relationships. These patterns enhance code reusability and promote flexibility by providing mechanisms for composing objects into more complex structures. Some common structural patterns include the Adapter pattern, which allows incompatible interfaces to work together through an adapter class, and the Composite pattern, which treats individual objects and compositions of those objects uniformly.
Behavioral patterns focus on how objects communicate with each other to accomplish tasks efficiently. These patterns address interactions between objects, encapsulate algorithms or behaviors within separate classes, and make systems more maintainable by decoupling implementation details from client code. Examples of behavioral patterns include the Observer pattern mentioned earlier, where objects can subscribe to events or changes in other objects, and the Strategy pattern, where different algorithms are encapsulated in separate classes allowing for interchangeable behavior at runtime.
By understanding these categories of design patterns and when to apply them appropriately in software development projects, developers can improve their code quality, reduce redundancy, enhance maintainability, and foster collaboration among team members. It is important to note that design patterns are not rigid rules but rather guidelines based on best practices accumulated over years of experience in software development. Therefore, it is crucial for developers to adapt these patterns according to project requirements while keeping in mind key principles such as encapsulation, abstraction, modularity, and separation of concerns.
In conclusion, design patterns are powerful tools that aid developers in solving common software design problems effectively. They provide reusable solutions that promote better code organization, modularity, flexibility, and maintainability. By applying appropriate design patterns in object-oriented programming projects, developers can create robust and scalable applications that are easier to understand, extend, and maintain over time.
Understanding Design Patterns
Design patterns are essential tools in object-oriented programming that provide solutions to recurring software design problems. By applying these patterns, developers can enhance the structure and flexibility of their code while improving maintainability and reusability. To illustrate the significance of design patterns, let us consider a hypothetical scenario where a team of developers is tasked with building an e-commerce website.
In this case, the development team faces multiple challenges such as managing different types of products, implementing various payment methods, handling user authentication, and ensuring scalability for future growth. Without proper design patterns, addressing each of these challenges individually could lead to complex and tightly coupled code.
To overcome these obstacles efficiently, developers can employ design patterns which offer proven solutions to similar problems encountered in software development. For instance, one commonly used pattern in this scenario would be the Factory Method pattern. This pattern allows for the creation of different product objects (e.g., books, electronics) without explicitly specifying their classes within client code.
Implementing design patterns offers several benefits:
- Enhanced Code Reusability: Design patterns promote modular designs that can be reused across different projects or even within the same project.
- Improved Maintainability: By adhering to established design principles, it becomes easier to understand and modify existing codebases.
- Increased Scalability: Design patterns facilitate extensibility by providing standardized approaches to adding new features or functionalities.
- Efficient Collaboration: Using common design patterns helps teams communicate more effectively as they share a common vocabulary and understanding of problem-solving techniques.
Pattern Name | Description | Example Usage |
---|---|---|
Singleton | Ensures only one instance of a class exists throughout the application’s lifecycle. | Database connections |
Observer | Allows objects to subscribe and receive notifications about changes or events from other objects. | Event-driven systems |
Strategy | Encapsulates interchangeable algorithms within a family, allowing them to be selected and used at runtime. | Sorting algorithms |
Decorator | Dynamically adds or modifies behavior of an object by wrapping it with other objects that possess the desired functionality. | Extending functionalities in graphic editors |
In conclusion, understanding design patterns is crucial for software developers aiming to create robust and maintainable code. By applying these proven solutions to recurring problems, development teams can avoid reinventing the wheel and improve collaboration while delivering high-quality software solutions. In the subsequent section, we will explore the benefits offered by incorporating design patterns into software development processes.
[Transition] Moving forward, let us examine the numerous benefits that arise from utilizing design patterns in software development, further highlighting their importance in creating efficient and scalable systems.
Benefits of Design Patterns
In the previous section, we explored the concept of design patterns and their significance in software development. Now, let us delve deeper into how these patterns are implemented to address real-world challenges faced by developers.
Consider the following scenario: a software development team is tasked with creating an e-commerce website that can handle a high volume of user traffic. To ensure efficient performance and maintainability of the codebase, the team decides to apply design patterns during the development process. One such pattern they might employ is the Singleton pattern, which allows for the creation of a single instance of a class throughout the application. By using this pattern, multiple instances of critical components, such as database connections or logging services, can be avoided, resulting in improved resource utilization and reduced complexity.
When it comes to practical implementation, there are various benefits associated with utilizing design patterns:
- Code reusability: Design patterns provide reusable solutions to common problems encountered during software development.
- Maintainability: Using established design patterns helps improve code readability and makes it easier to understand and modify existing systems.
- Scalability: Applying appropriate design patterns from the outset ensures that software architectures are adaptable and capable of accommodating future growth.
- Collaboration: Design patterns serve as a common language among developers, enabling effective communication and collaboration within teams.
To further illustrate the impact of design patterns on software development practices, consider Table 1 below showcasing four commonly used design patterns along with their key characteristics:
Design Pattern | Description |
---|---|
Observer | Defines one-to-many dependency between objects |
Factory | Provides an interface for creating objects without specifying |
their concrete classes | |
Strategy | Enables dynamic selection of algorithms at runtime |
Decorator | Allows adding new functionality to an object dynamically |
Table 1: Commonly Used Design Patterns
By incorporating these tried-and-tested approaches into software development, developers can enhance the quality and reliability of their code.
Commonly Used Design Patterns
Benefits of Design Patterns in Software Development
In the previous section, we explored the benefits of using design patterns in software development. Now, let us delve further into commonly used design patterns and understand their significance in object-oriented programming.
To illustrate the importance of design patterns, consider a hypothetical case study involving an e-commerce website. The developers faced the challenge of implementing a shopping cart feature that could handle multiple products with varying quantities. By applying the Singleton design pattern, they were able to ensure that only one instance of the shopping cart existed throughout the application. This prevented data inconsistencies and improved overall system performance.
When it comes to utilizing design patterns effectively, there are several key points worth considering:
- Code Reusability: Design patterns promote code reusability by providing standardized solutions to certain recurring problems. This allows developers to save time and effort by leveraging pre-existing designs rather than starting from scratch.
- Scalability: Design patterns enable applications to scale efficiently as they provide flexible structures that can accommodate changing requirements without significant modifications to existing code.
- Maintainability: With well-defined architectural principles, design patterns enhance code maintainability by promoting modularity and separation of concerns. This makes it easier for developers to identify and isolate issues when making updates or fixing bugs.
- Collaboration: Using established design patterns facilitates collaboration among team members as it establishes a common language and understanding within the development community.
Let’s now explore some commonly used design patterns through a table format below:
Pattern | Description | Example Use Cases |
---|---|---|
Factory Method | Defines an interface for creating objects but lets subclasses decide which class to instantiate | Creating different types of payment gateways |
Observer | Establishes a one-to-many relationship between objects so that changes in one notify others | Implementing event-driven systems |
Strategy | Encapsulates interchangeable algorithms, allowing them to be easily replaced or extended | Implementing different sorting algorithms |
Decorator | Dynamically adds new behavior to an object without altering its structure | Adding additional functionality to a text editor |
In summary, design patterns provide a structured approach to software development, offering numerous benefits such as code reusability, scalability, maintainability, and improved collaboration among developers. By incorporating commonly used design patterns into their projects, developers can create robust and flexible applications that are easier to manage and enhance.
Moving forward, let’s explore the next section on Creational Design Patterns and how they contribute to effective software development.
Creational Design Patterns
Design Patterns: Software Development in Object-Oriented Programming
Commonly Used Design Patterns have been discussed extensively in the previous section, emphasizing their importance and applicability within software development. Now, let us delve into another significant category of design patterns known as Creational Design Patterns. To illustrate the concept, imagine a scenario where a team is developing an e-commerce platform from scratch.
Consider a hypothetical case study where this team wants to implement a shopping cart feature. They need to ensure that only one instance of the cart exists throughout the system, enabling users to add items while maintaining consistency across different parts of the application. In such cases, they can employ creational design patterns like Singleton or Prototype.
Creational Design Patterns typically serve three main purposes:
- Provide ways to create objects without explicitly specifying their exact classes.
- Encapsulate object creation logic, making it more flexible and reusable.
- Ensure that only specific instances are created based on predefined criteria.
These patterns incorporate various techniques and strategies to achieve these goals. Some commonly used creational design patterns include:
- Singleton Pattern: Ensures that there is only one instance of a class available throughout the system.
- Factory Method Pattern: Defines an interface for creating objects but allows subclasses to decide which class to instantiate.
- Abstract Factory Pattern: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder Pattern: Separates the construction of complex objects from their representation, allowing step-by-step assembly with different configurations.
By employing these creational design patterns effectively, developers can enhance code maintainability and flexibility while reducing coupling between components. This not only improves overall software quality but also contributes towards efficient collaboration among diverse teams working on large-scale projects.
Understanding both Creational and Structural Design Patterns is essential for software developers to design robust and scalable applications that align with industry best practices.
Structural Design Patterns
Section H2: ‘Structural Design Patterns’
As we delve deeper into the world of design patterns, it is important to explore another crucial category known as structural design patterns. These patterns focus on organizing objects and classes in a way that enhances their relationships and simplifies system structure. To illustrate this concept, let’s consider an example scenario involving a large e-commerce platform.
Imagine you are working for an online marketplace where sellers can showcase their products to potential buyers. One common challenge faced by such platforms is efficiently managing product categories and subcategories. By utilizing the Composite pattern from the structural design patterns repertoire, our hypothetical team could simplify this process. The Composite pattern allows us to treat individual objects (products) and collections of those objects (product categories) uniformly, enabling seamless navigation through the hierarchy.
To further understand the significance of structural design patterns, let’s examine some key benefits they offer:
- Enhanced code reusability: Structural design patterns promote reusable code components by facilitating flexible object composition.
- Improved maintainability: By decoupling complex systems into smaller modules, these patterns make maintenance and updates more manageable.
- Increased extensibility: With well-designed structures, adding new features or modifying existing ones becomes less prone to errors and complications.
- Efficient resource utilization: Effective structuring ensures optimal usage of resources such as memory, processing power, and network bandwidth.
Pattern Name | Description | Example Usage |
---|---|---|
Adapter | Converts interfaces between incompatible classes allowing them to work together | Converting data formats/APIs |
Decorator | Dynamically adds functionality to objects at runtime | Adding additional behavior to core classes |
Facade | Provides a simplified interface for a subsystem or group of related interfaces | Simplifying interactions with complex systems |
Proxy | Provides a surrogate or placeholder for another object to control access, add functionality | Controlling access to sensitive information |
In conclusion, structural design patterns play a crucial role in enhancing the organization and relationships of objects within a software system. By utilizing these patterns effectively, developers can improve code reusability, maintainability, extensibility, and resource utilization.
Transitioning into the subsequent section: Continuing our exploration of design patterns, let’s now turn our attention towards an equally important aspect – behavioral design patterns. These patterns emphasize how objects interact with each other and encapsulate complex communication logic within them.
Behavioral Design Patterns
Section H2: Behavioral Design Patterns
Transitioning from the previous section on structural design patterns, we now delve into behavioral design patterns. These patterns focus on the interactions between objects and how they communicate with each other to achieve desired behaviors within a software system.
To illustrate the importance of behavioral design patterns, let’s consider an example scenario where a team of developers is working on creating an e-commerce website. One of the key functionalities required for this website is a shopping cart feature that allows users to add items, remove items, and calculate the total price. In order to implement this functionality efficiently and maintainable, the team can leverage various behavioral design patterns.
One such pattern is the Observer pattern which facilitates communication between objects in a loosely coupled manner. By applying this pattern, the shopping cart object can observe changes in item quantities or prices and automatically update itself accordingly. This ensures that any modifications made to individual items are accurately reflected in the overall calculations performed by the shopping cart.
When considering behavioral design patterns, it is useful to keep in mind their potential benefits. Here are some advantages of using these patterns:
- Improved code reusability and flexibility.
- Enhanced modularity and maintainability.
- Better separation of concerns, leading to more understandable code.
- Facilitation of unit testing and debugging processes.
These benefits ultimately contribute to increased productivity and efficiency in software development projects. To further emphasize their significance, consider the following table showcasing four popular behavioral design patterns along with their corresponding descriptions:
Pattern | Description |
---|---|
Chain of Responsibility | Allows multiple objects to handle a request sequentially until one object successfully handles it or all have been exhausted without success. |
Command | Encapsulates a request as an object, allowing clients to parameterize clients with different requests, queue or log requests’ execution etc. |
Iterator | Provides a way to access elements of an aggregate object sequentially without exposing its underlying representation. |
State | Allows an object to alter its behavior when its internal state changes, thus appearing as if it is changing its class. |
In conclusion, behavioral design patterns play a crucial role in ensuring effective communication and interaction between objects within a software system. By utilizing these patterns appropriately, developers can achieve code that is more reusable, maintainable, and modular. The Observer pattern presented earlier provides just one example of how leveraging behavioral design patterns can enhance the functionality and flexibility of software systems.