Inheritance: Software Development in Object-Oriented Programming
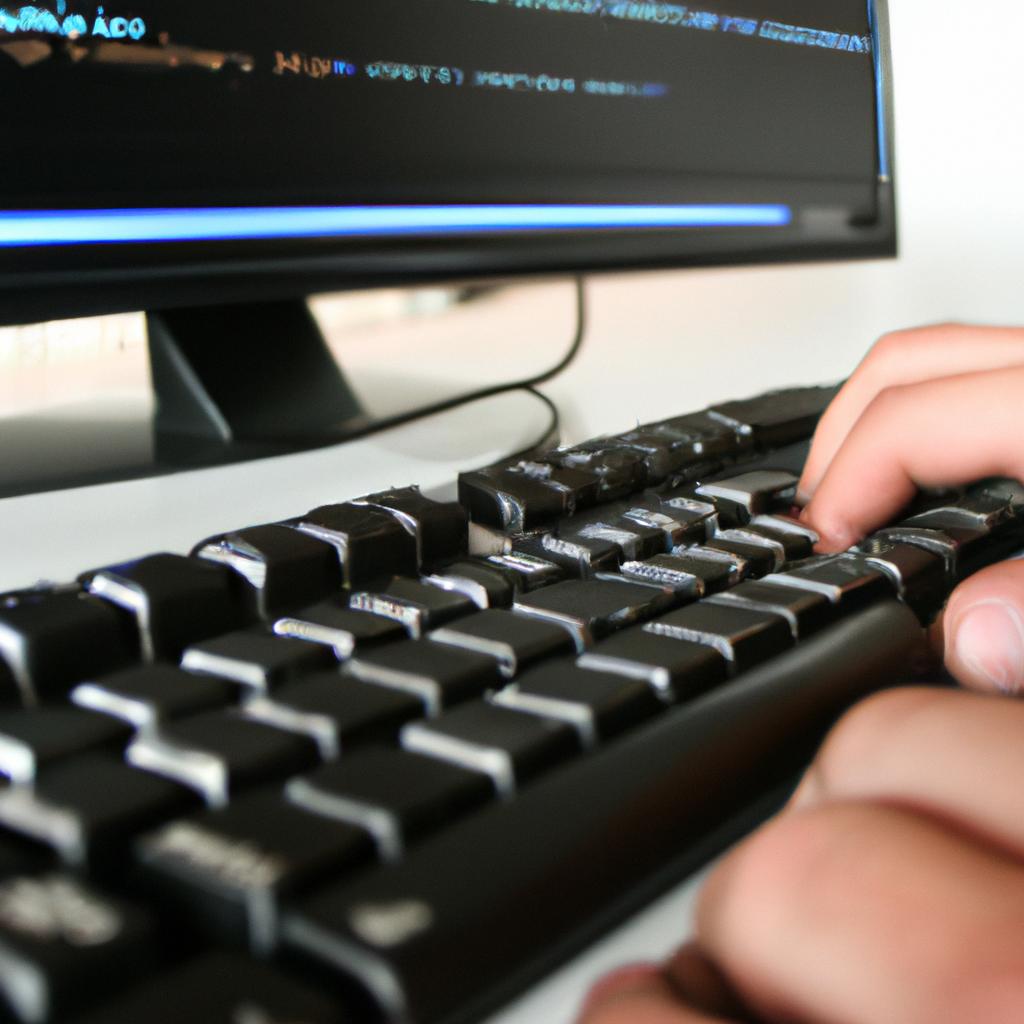
In the field of software development, inheritance is a crucial concept in object-oriented programming that allows for the creation and organization of complex code structures. By enabling classes to inherit properties and methods from other classes, developers can efficiently reuse existing code, promote modular design, and enhance overall program functionality. For instance, consider a hypothetical scenario where a company develops an e-commerce application. The application requires different types of products such as electronics, clothing, and books. Instead of writing separate code for each product type, inheritance allows developers to create a base class representing common attributes like price and description, which can be inherited by specific product classes.
Object-oriented programming emphasizes the concepts of encapsulation, abstraction, polymorphism, and most importantly, inheritance. Inheritance plays a significant role in promoting code reusability by allowing objects to acquire characteristics from parent objects or superclasses. It enables developers to establish hierarchical relationships between classes based on shared attributes and behaviors while providing flexibility for customization through subclassing. This article aims to explore the fundamentals of inheritance in software development within the context of object-oriented programming principles. By examining its benefits and applications in real-world scenarios such as building robust software systems or developing scalable frameworks, we aim to provide insight into how inheritance can significantly impact the efficiency and effectiveness of software development processes.
One of the primary benefits of inheritance is code reuse. By creating a base class with common attributes and methods, developers can avoid duplicating code across different classes. This not only reduces the amount of code that needs to be written but also makes it easier to maintain and update the application in the long run. For example, if there is a change in the pricing logic for all products, developers can modify the code in one place (the base class) instead of updating each individual product class.
Inheritance also promotes modular design and enhances program functionality. It allows for the creation of specialized classes that inherit properties and behaviors from more general classes. This hierarchical structure makes it easier to organize and manage complex systems by breaking them down into smaller, more manageable components. For instance, in an e-commerce application, developers can create separate classes for electronics, clothing, and books that inherit from a common product class. Each specific product class can then have its own unique attributes or methods while still benefiting from the shared functionality provided by the base class.
Furthermore, inheritance enables polymorphism, which is another important concept in object-oriented programming. Polymorphism allows objects of different types to be treated as instances of a common superclass. This flexibility allows for writing generic code that can work with objects of different subclasses interchangeably. In our e-commerce example, this means that we can write code that works with any type of product without needing to know specifically whether it’s electronics, clothing, or books.
Overall, inheritance plays a crucial role in software development by facilitating code reuse, promoting modularity and scalability, enhancing program functionality through polymorphism, and improving overall efficiency in building complex systems. It is an essential concept for developers to understand and utilize effectively when designing and implementing object-oriented software solutions.
Benefits of Inheritance in Object-Oriented Programming
In the world of software development, inheritance plays a crucial role in the implementation of object-oriented programming (OOP) principles. By allowing classes to inherit properties and behaviors from other classes, inheritance facilitates code reuse, promotes modularity, and enhances extensibility. These benefits have made it an indispensable component in modern software engineering practices.
To illustrate the significance of inheritance, let us consider a hypothetical scenario where we are developing a banking application. In this system, various types of bank accounts such as savings account, current account, and fixed deposit account need to be implemented. Without utilizing inheritance, each type of account would require its own separate class with redundant code for common functionalities like balance checking or transaction handling. However, by employing inheritance, we can create a generic account class that contains these shared attributes and methods. Subsequently, specific account types can inherit from this base class while also having their unique characteristics. This approach not only simplifies code maintenance but also improves overall efficiency.
The advantages provided by inheritance extend beyond mere reduction in redundancy. They include:
- Code Reusability: Inheritance allows developers to reuse existing code without duplicating it across multiple classes. It enables them to build upon already defined behaviors and attributes in parent classes within child classes.
- Modularity: With inheritance, complex systems can be broken down into smaller modules encapsulated within individual classes. Each module represents a distinct behavior or functionality related to the problem domain.
- Extensibility: Inheritance provides flexibility for future modifications or enhancements to the software system. New features can be easily added by extending existing classes or introducing new derived classes.
- Simplification: The use of hierarchical relationships between classes through inheritance simplifies program understanding and maintenance by providing a clear structure and organization.
Advantages | Description |
---|---|
Code Reusability | Allows for the reuse of existing code, reducing redundancy and improving development efficiency. |
Modularity | Enables the division of complex systems into smaller, encapsulated modules for better management. |
Extensibility | Provides flexibility to add new features or functionalities by extending existing classes. |
Simplification | Offers a structured and organized approach to program design, facilitating understanding and maintenance. |
Understanding the concept of inheritance is crucial in object-oriented programming as it forms the foundation upon which more advanced concepts are built. The next section will delve deeper into this fundamental aspect, exploring its various types and their implications within software development contexts.
Inheritance allows for code reusability, modularity, extensibility, and simplification in software engineering practices. By employing this powerful mechanism, developers can efficiently build complex systems while maintaining ease of maintenance and scalability. Understanding how inheritance works serves as a stepping stone towards mastering other essential principles of object-oriented programming.
Understanding the Concept of Inheritance
Building upon the previous discussion on the benefits of inheritance in object-oriented programming, let us now explore a concrete example that highlights how this concept can be applied effectively. Consider a scenario where a software development company is tasked with creating an inventory management system for a large retail chain. By utilizing inheritance, the developers can design and implement a robust and scalable solution that meets the specific requirements of their client.
One significant benefit of using inheritance in this context is code reusability. The developers can create a base class, such as “Product,” which contains common attributes and methods shared by all types of products sold by the retail chain, including clothing, electronics, and groceries. Subclasses can then inherit from this base class to define specialized product types with additional properties or behaviors unique to each category. This approach not only saves time but also promotes consistency throughout the codebase.
Additionally, inheritance facilitates easier maintenance and updates. Suppose the retail chain decides to introduce a new type of product called “beauty products” into its inventory management system. With proper use of inheritance, adding support for this new product type becomes straightforward: it involves defining a new subclass that inherits from the existing “Product” base class and includes any necessary modifications or extensions specific to beauty products.
- Increased efficiency through code reuse.
- Improved maintainability due to modularized structure.
- Enhanced flexibility when introducing new features.
- Streamlined collaboration among developers working concurrently on different parts of the project.
Furthermore, we present below a table showcasing some key benefits offered by inheritance:
Benefit | Description |
---|---|
Code Reusability | Reduces redundancy and improves overall productivity |
Modularity | Simplifies testing, debugging, and maintenance processes |
Scalability | Enables easy expansion without disrupting existing functionality |
Encapsulation and Abstraction | Promotes clean code design and separation of concerns |
As we delve deeper into the world of software development, it becomes evident that understanding inheritance is crucial for writing efficient and maintainable code. In the subsequent section, we will explore different types of inheritance commonly employed in software development, further expanding our knowledge in this area.
Types of Inheritance in Software Development
In the previous section, we discussed the concept of inheritance in software development. Now, let’s delve deeper into different types of inheritance that are commonly used in object-oriented programming.
Before we dive into the specifics, consider this hypothetical example: imagine you are developing a banking application where various account types need to be implemented such as savings accounts, checking accounts, and credit card accounts. While each type has its unique features and functionalities, they also share some common attributes like account number, balance, and customer information. Implementing these shared features individually for each account type would result in redundant code and decreased maintainability. However, by utilizing inheritance, we can create a base class called “Account” with all the common attributes and behaviors. Then, specific account types can inherit from this base class and add their own additional properties or methods as needed. This way, code reusability is increased while maintaining flexibility.
There are several types of inheritance that can be employed based on the requirements of a software project. Let’s explore four main categories:
- Single Inheritance: In single inheritance, a derived class inherits properties and methods from only one base class.
- Multiple Inheritance: As the name suggests, multiple inheritance allows a derived class to inherit from two or more base classes simultaneously.
- Multilevel Inheritance: Multilevel inheritance occurs when a derived class inherits properties and methods not just from one but from another derived class as well.
- Hierarchical Inheritance: Hierarchical inheritance involves multiple derived classes inheriting from a single base class independently.
To illustrate these concepts further, refer to the following table:
Types of Inheritance | Explanation |
---|---|
Single | A child inherits characteristics solely from one parent class which results in an organized hierarchy within the program structure |
Multiple | A child inherits characteristics from multiple parent classes, allowing for a more diverse and versatile program structure |
Multilevel | A child class inherits properties and methods not only from its direct parent but also from the ancestor classes above it |
Hierarchical | Multiple child classes inherit properties and methods from a single base class independently, providing flexibility in the program design |
In summary, inheritance is an essential concept in object-oriented programming that allows for code reuse, increased modularity, and improved maintainability. By utilizing different types of inheritance based on project requirements, developers can construct efficient and structured software systems.
Key Principles of Inheritance
In the previous section, we explored the concept of inheritance in software development. Now let’s delve deeper into different types of inheritance that are commonly used in object-oriented programming.
One example of inheritance is a scenario where you have a base class called “Animal” and derived classes such as “Dog,” “Cat,” and “Bird.” Each derived class inherits properties and behaviors from the base class but can also add additional features specific to each animal type. For instance, while all animals may have a method called “eat()” inherited from the Animal base class, individual species like dogs or cats may have their own unique methods like “bark()” or “meow()”.
To better understand the various types of inheritance, consider these key principles:
- Single Inheritance: This involves inheriting properties and behaviors from only one parent class. It allows for a simple hierarchy with clear relationships between classes.
- Multiple Inheritance: With this type of inheritance, a child class can inherit attributes and functionalities from more than one parent class. While it offers flexibility, it can lead to ambiguity if two parents share similar methods or attributes.
- Multilevel Inheritance: Here, a derived class becomes the base class for another derived class. This creates a hierarchical structure with levels of subclasses inheriting characteristics from their respective superclasses.
- Hierarchical Inheritance: This occurs when multiple classes inherit properties and methods from a single base or parent class. The superclass acts as the common source for all its subclasses.
The table below summarizes these types of inheritance:
Type | Description |
---|---|
Single Inheritance | Inherits properties and behaviors from only one parent class |
Multiple Inheritance | Inherits attributes and functionalities from more than one parent |
Multilevel Inheritance | A derived class becomes the base class for another derived class |
Hierarchical Inheritance | Multiple classes inherit from a single base or parent class |
Understanding the different types of inheritance is crucial when designing object-oriented software systems. It allows developers to create efficient and scalable code by reusing existing functionality while also extending it as needed.
Moving forward, let’s explore some common mistakes to avoid in inheritance that can potentially lead to design flaws and inefficiencies in software development.
Common Mistakes to Avoid in Inheritance
In the previous section, we discussed the key principles of inheritance in object-oriented programming. Now, let’s delve into some common mistakes that programmers should avoid when using inheritance.
Imagine a scenario where a software development team is working on a project to create an e-commerce platform. One developer decides to use inheritance to implement different types of payment methods such as credit card and PayPal. However, they make the mistake of creating a deep hierarchy of classes for each payment method, resulting in complex and tightly coupled code.
To help you navigate potential pitfalls and improve your understanding of inheritance, here are some important points to consider:
- Avoid excessive class hierarchies: It is crucial not to create overly complicated class hierarchies with numerous levels of inheritance. This can lead to code that is difficult to understand, maintain, and extend. Instead, focus on maintaining simplicity by keeping the hierarchy shallow and concise.
- Watch out for improper overriding: When inheriting from a base class, it may be necessary to override certain methods or properties. However, care must be taken not to accidentally change their behavior unintentionally. Always double-check whether the overridden members adhere to the intended design and functionality.
- Beware of violating Liskov Substitution Principle: The Liskov Substitution Principle states that objects of derived classes should be able to substitute objects of their base classes without affecting program correctness. Violating this principle can introduce subtle bugs and unexpected behaviors within your system.
- Avoid relying too heavily on implementation details: Inheritance allows us to reuse existing code, but it also introduces dependencies between classes. Be cautious about relying too much on specific implementations from parent classes as changes made there might have unintended consequences down the line.
To further illustrate these concepts visually, take a look at the following table showcasing examples of common mistakes in inheritance alongside their negative impacts:
Mistake | Negative Impact |
---|---|
Deep class hierarchies | Increased code complexity and maintenance difficulties |
Improper method overriding | Unintended changes in behavior |
Violation of Liskov Substitution Principle | Runtime errors and unexpected program behavior |
Overreliance on implementation details | Brittle code that is prone to breaking when modifications occur |
In summary, by avoiding these common mistakes, you can ensure a more robust and maintainable software system.
Section: Best Practices for Implementing Inheritance
In the previous section, we discussed some common mistakes that developers should avoid when working with inheritance in object-oriented programming. Now, let’s delve into best practices for implementing inheritance effectively.
To illustrate these best practices, let’s consider a hypothetical scenario where a software development team is tasked with creating a banking application. The team decides to use inheritance to model different types of bank accounts, such as savings accounts and checking accounts.
-
Clearly define the hierarchy: When utilizing inheritance, it is crucial to establish a clear and well-defined class hierarchy. This means identifying the relationships between classes and ensuring that each subclass accurately represents its superclass. For example, in our banking application case study, the SavingsAccount class should inherit from the Account class since all savings accounts are also considered general bank accounts.
-
Use abstraction and encapsulation: Abstraction allows us to focus on essential properties and behaviors while hiding unnecessary details. By leveraging abstract classes or interfaces, we can enforce certain methods across multiple subclasses without specifying their implementation details explicitly. Encapsulation ensures that data is kept private within each class, promoting code reusability and minimizing dependencies among classes.
-
Practice method overriding carefully: Method overriding enables subclasses to provide their own implementation of inherited methods from superclasses. However, it is vital to exercise caution when overriding methods as it can lead to unintended consequences if not done correctly. Developers should thoroughly understand how polymorphism works in order to ensure proper behavior throughout the inheritance hierarchy.
Emotional Bullet Point List
The following bullet points highlight the emotional impact of implementing effective inheritance practices:
- Simplifies code maintenance
- Enhances flexibility and extensibility
- Promotes code reuse and modularity
- Reduces development time by eliminating redundant coding efforts
Emotional Impact | Benefits |
---|---|
Simplifies | Code maintenance |
Enhances | Flexibility and extensibility |
Promotes | Code reuse and modularity |
Reduces | Development time |
In conclusion, implementing inheritance correctly is crucial for developing robust and maintainable object-oriented software. By clearly defining class hierarchies, leveraging abstraction and encapsulation, and carefully practicing method overriding, developers can ensure a solid foundation for their codebase. Following these best practices not only leads to efficient development but also improves the overall quality of the software product.