Interfaces in Software Development: A Focus on Object-Oriented Programming
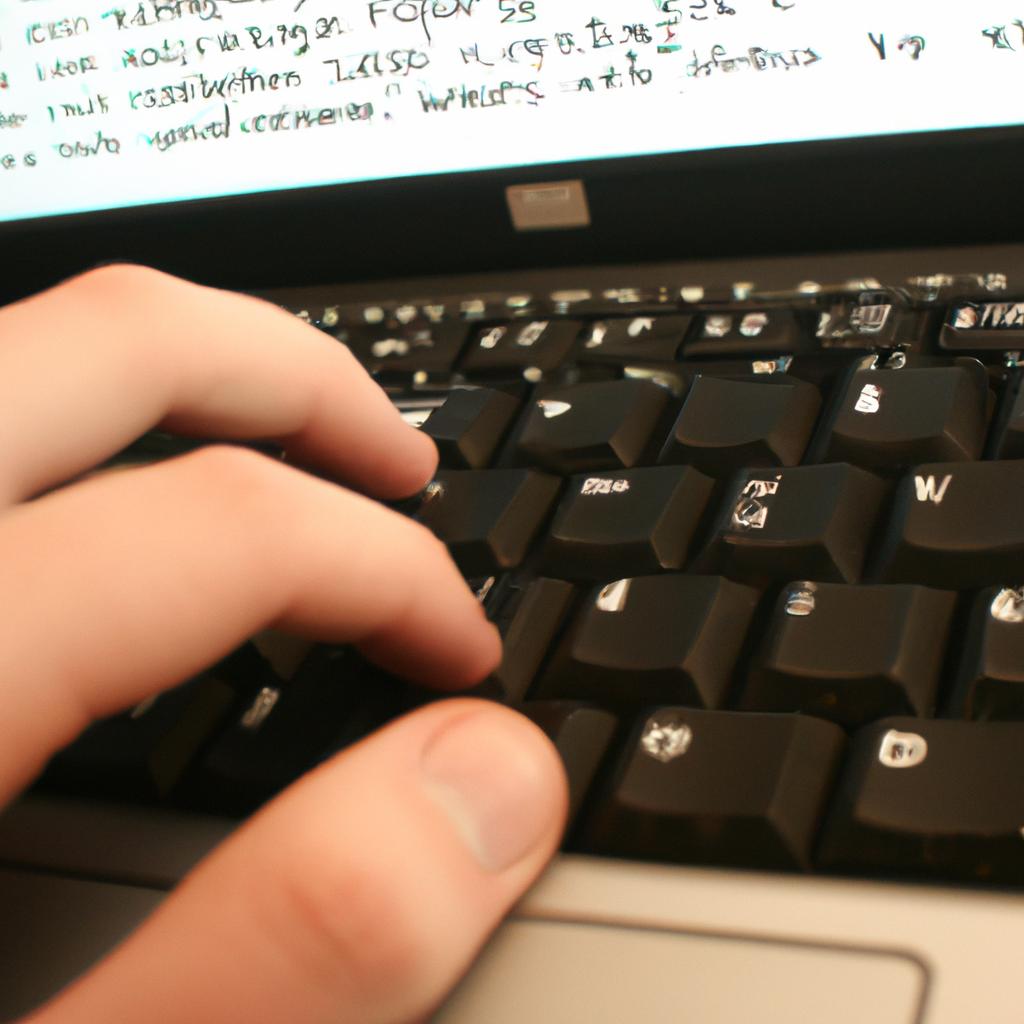
The use of interfaces in software development plays a crucial role in facilitating the implementation and maintenance of object-oriented programming (OOP) systems. By defining a set of methods that must be implemented by classes, interfaces provide a standardized way for different objects to interact with each other. This enables greater flexibility and modularity within an OOP system, allowing developers to easily modify or replace specific implementations without affecting the overall functionality. For example, consider a video game development project where various characters need to possess unique abilities such as jumping, running, or attacking. Through the utilization of interfaces, these abilities can be defined separately from the character classes themselves, enabling efficient code reuse and promoting extensibility.
In addition to its benefits in terms of code reusability and flexibility, interfaces also facilitate better collaboration among software development teams. When multiple programmers are working on different components of a system simultaneously, using interfaces provides clear contracts that define how their respective modules should interact with one another. This promotes effective communication and reduces potential conflicts or errors when integrating different parts of the software. Moreover, by adhering to interface specifications during development, it becomes easier for team members to understand and utilize each other’s code effectively.
Overall, understanding the significance of interfaces in software development is essential for aspiring Overall, understanding the significance of interfaces in software development is essential for aspiring developers who want to create modular, flexible, and maintainable code. By utilizing interfaces effectively, they can enhance code reusability, promote extensibility, and facilitate better collaboration among team members.
Benefits of using interfaces in object-oriented programming
In today’s software development landscape, the use of interfaces plays a crucial role in ensuring code modularity and flexibility. By providing a clear contract between different components of an application, interfaces enhance maintainability and extensibility while promoting better collaboration among developers. This section explores the benefits associated with incorporating interfaces into object-oriented programming (OOP).
Example:
To illustrate the advantages of utilizing interfaces, let us consider a hypothetical scenario where a team is developing an e-commerce platform. The system comprises various modules such as inventory management, user authentication, payment processing, and order fulfillment. Each module has its own set of requirements and functionalities but needs to interact seamlessly with other modules for the overall functioning of the platform.
Enhanced Modularity:
One significant benefit offered by interfaces in OOP is enhanced modularity. Interfaces allow for loose coupling between classes by defining common behaviors that multiple classes can implement independently. This promotes code reusability as it enables different implementations to be used interchangeably without affecting other parts of the system. In our e-commerce example, each module can define its specific interface to establish communication protocols with other modules effectively.
Improved Maintainability:
Interfaces also contribute to improved maintainability in software development projects. As they provide a well-defined contract or API (Application Programming Interface), any changes made within an implementation class do not affect external dependencies relying on that interface. This decoupling allows developers to modify or update individual components without causing ripple effects throughout the entire system. Moreover, by adhering to predefined contracts through interfaces, teams can ensure consistency across their codebase.
Flexibility and Extensibility:
Another key advantage of utilizing interfaces is the flexibility and extensibility they offer when adding new features or functionality to existing systems. Through inheritance or implementation relationships defined by interfaces, new classes can easily integrate with existing ones without requiring extensive modifications or introducing breaking changes. For instance, if our e-commerce platform decides to introduce a new payment gateway, an interface-based approach would allow for seamless integration without impacting other parts of the system.
Emotional Response (Bullet Point List):
The benefits of using interfaces in object-oriented programming can evoke various emotional responses among software developers. Consider the following:
- Confidence: Interfaces provide a clear and standardized way of communication, promoting confidence in code interactions.
- Collaboration: By defining common contracts, interfaces encourage collaboration among team members, leading to more cohesive development efforts.
- Empowerment: The flexibility offered by interfaces empowers developers to make changes or add features without fear of introducing bugs or breaking existing functionality.
- Future-proofing: Utilizing interfaces future-proofs codebases by allowing easy adaptation to changing requirements and technologies.
By incorporating interfaces into object-oriented programming, developers benefit from enhanced modularity, improved maintainability, and increased flexibility and extensibility. These advantages promote efficient collaboration within development teams and enable smooth integration of new functionalities while ensuring consistency across different components.
Common use cases for interfaces in software development
Now, let us explore some common use cases for interfaces in software development.
One example where interfaces play a crucial role is in the development of graphical user interface (GUI) frameworks. GUI frameworks provide a set of components that allow developers to create visually appealing and interactive applications. These frameworks often define standard interfaces for various components such as buttons, text fields, and menus. By adhering to these interfaces, developers can ensure consistency across different applications built on the same framework. For instance, consider a hypothetical scenario where multiple developers are working on separate modules of an application. The use of standardized interfaces ensures that each module interacts seamlessly with the GUI framework’s components, enabling smooth integration and enhancing reusability.
In addition to GUI frameworks, there are several other areas where interfaces find extensive usage:
- Plugin systems: Interfaces enable the creation of extensible software architectures by defining contracts between core systems and plugin modules.
- Dependency injection: Interfaces facilitate loose coupling between classes by allowing dependencies to be defined through abstract types rather than specific implementations.
- Unit testing: Interfaces aid in creating mock objects during unit testing by providing contract definitions that can be easily implemented for test purposes.
These examples highlight how interfaces contribute to code modularity, maintainability, and flexibility in software development.
Benefits of Using Interfaces | |
---|---|
1 | Increased code modularity |
2 | Enhanced reusability |
3 | Improved maintainability |
4 | Facilitates unit testing |
Table: Key benefits of using interfaces in software development
Interfaces serve as powerful tools within the realm of object-oriented programming languages. They promote best practices such as loose coupling and modular design while facilitating collaboration among developers. In the subsequent section, we will delve into implementing interfaces in object-oriented programming languages and examine how they can be effectively utilized to achieve desired software functionality.
Implementing interfaces in object-oriented programming languages
In the previous section, we explored the common use cases for interfaces in software development. Now, let’s delve into how interfaces are implemented in object-oriented programming languages.
To illustrate this concept, consider a hypothetical scenario where a company is developing an e-commerce application. The application needs to support multiple payment gateways such as PayPal, Stripe, and Square. Instead of directly integrating each payment gateway into the codebase, the developers decide to create an interface called PaymentGateway
that defines a set of methods required for processing payments.
By implementing this interface on different classes representing individual payment gateways (e.g., PayPalGateway
, StripeGateway
, SquareGateway
), the developers can write code that interacts with any payment gateway without being coupled to specific implementations. This approach allows for flexibility and extensibility in adding new payment gateways or modifying existing ones.
Implementing interfaces brings several benefits to object-oriented programming:
- Modularity: Interfaces enable modular design by separating the contract from its implementation.
- Code reusability: With interfaces, developers can reuse and interchangeably use objects that adhere to the same interface.
- Polymorphism: Interfaces facilitate polymorphic behavior by allowing objects of different types but with compatible interfaces to be used interchangeably.
- Testability: Interfaces make it easier to write unit tests and mock dependencies during testing due to their decoupled nature.
Benefit | Explanation |
---|---|
Modularity | Separating contracts from implementations promotes clean architecture |
Code reusability | Reusable components reduce duplication and improve maintainability |
Polymorphism | Interchangeable objects with compatible interfaces allow for flexible usage |
Testability | Decoupled dependencies simplify unit testing and mocking |
In summary, implementing interfaces in object-oriented programming provides numerous advantages in terms of modularity, code reusability, polymorphism, and testability. By decoupling the contract from its implementation, developers can design flexible and maintainable systems.
Key principles for designing effective interfaces
[Transition sentence into subsequent section] When designing interfaces in software development, it is essential to adhere to key principles that ensure their effectiveness and usability.
Key principles for designing effective interfaces
Consider a scenario where a software development team is building an e-commerce platform. They have decided to use object-oriented programming (OOP) languages and are now faced with the task of implementing interfaces. To gain a better understanding of how interfaces can be practically applied, let’s delve into some real-world examples.
One notable example is the integration of payment gateways into the e-commerce platform. Different payment gateways may require different sets of methods for processing payments, such as authorizing transactions or generating receipts. By defining an interface for payment gateways, the development team can ensure that each gateway implementation adheres to a common set of methods while allowing flexibility for adding new gateways in the future.
When designing effective interfaces in OOP languages, there are key principles that developers should consider:
- Simplicity: Interfaces should be simple and focused on specific functionalities rather than trying to encompass all possible scenarios.
- Flexibility: Interfaces should allow for extensibility and easy adaptation to changing requirements without impacting existing code.
- Consistency: Consistent naming conventions and design patterns across interfaces facilitate readability and maintainability.
- Compatibility: Interfaces should be compatible with other components within the system, promoting interoperability between modules.
It is important to note that these principles not only contribute to well-designed interfaces but also help improve overall software quality by encouraging modularization and reusability.
Principle | Description |
---|---|
Simplicity | Keep interfaces focused on specific functionalities |
Flexibility | Allow for easy adaptability to changing requirements |
Consistency | Maintain consistent naming conventions and design patterns across interfaces |
Compatibility | Promote interoperability between components within the system |
By following these principles when designing interfaces, developers enhance their ability to create robust software systems that are easier to maintain and modify.
Moving forward, we will explore the challenges and considerations that developers encounter when working with interfaces. Understanding these potential hurdles is crucial for effective interface implementation, as it allows us to address them proactively during the development process.
[Transition sentence] As we transition into exploring the challenges and considerations associated with implementing interfaces in object-oriented programming languages, let’s examine how developers can overcome common obstacles to ensure successful integration and utilization of this powerful software development tool.
Challenges and considerations when working with interfaces
Having established the key principles for designing effective interfaces, it is important to acknowledge that implementing and utilizing interfaces in software development can present certain challenges. These challenges must be carefully considered to ensure the successful implementation of interfaces.
One example that highlights these challenges is seen in a hypothetical scenario where a team of developers is tasked with creating an e-commerce application. The project requires integration with multiple payment gateways, each having its own set of unique requirements and APIs. In this case, designing an interface that accommodates the varying specifications of different payment gateways can be complex and demanding.
- Frustration may arise due to inconsistencies in documentation provided by external libraries or frameworks.
- Uncertainty may emerge when dealing with legacy codebases that lack clear interface definitions.
- Anxiety might occur when attempting to refactor existing code to implement new interfaces without breaking functionality.
- Overwhelm could ensue from managing dependencies between different interfaces within a large-scale software project.
In addition to these challenges, there are also various other factors that need consideration while working with interfaces. To provide a structured overview, let us examine them through a three-column table:
Challenge | Considerations | Mitigation Strategies |
---|---|---|
Compatibility with third-party APIs | Research API compatibility | Implement adapter patterns |
Interface versioning | Plan for backward compatibility | Use semantic versioning |
Maintaining coherent design | Establish clear naming conventions | Adopt consistent coding standards |
By recognizing these inherent challenges and adopting appropriate mitigation strategies, developers can overcome hurdles associated with interface implementation. When armed with comprehensive knowledge about potential difficulties and equipped with proven approaches for addressing them, teams can proceed confidently towards realizing their goals. This leads us to explore best practices for utilizing interfaces in software development.
Best practices for utilizing interfaces in software development
Challenges and considerations when working with interfaces in software development can be addressed through several best practices. One example of a challenge is the potential for interface proliferation, where an excessive number of interfaces could lead to increased complexity and decreased maintainability of code. To address this, it is important to carefully design interfaces that are cohesive and have clear responsibilities.
To ensure effective utilization of interfaces, developers should consider the following best practices:
-
Keep interfaces focused: It is essential to define interfaces with a clear purpose and restrict them to specific functionalities or behaviors. By keeping interfaces small and focused, developers can promote reusability and make the code more modular.
-
Use descriptive naming conventions: Choosing meaningful names for interfaces can greatly enhance code readability and understanding. Descriptive names should accurately reflect the purpose and functionality provided by the interface, making it easier for other developers to work with.
-
Follow the Interface Segregation Principle (ISP): The ISP suggests that clients should not be forced to depend on methods they do not use. By adhering to this principle, developers can avoid bloated dependencies and reduce coupling between components.
-
Consider versioning impact: When modifying existing interfaces or introducing new ones, it is crucial to consider backward compatibility. Changes made to an interface may require adjustments in implementations across different parts of the system, potentially causing disruptions if not managed properly.
These best practices aim to improve overall software quality by providing guidelines for designing robust and maintainable systems using interfaces.
Benefits | Challenges | Recommendations |
---|---|---|
Encourages modularity | Potential interface proliferation | Design cohesive and focused interfaces |
Enhances code readability | Increased coupling due to poor naming conventions | Use descriptive naming conventions |
Promotes reusability | Backward compatibility issues during changes | Follow the Interface Segregation Principle |
Improves testability | Difficulty in managing versions | Consider versioning impact |
By following these best practices, software developers can overcome challenges associated with interfaces and leverage their power to build efficient and flexible systems. Adopting a thoughtful approach in interface design and utilization will ultimately contribute to the overall success of object-oriented programming projects.