Encapsulation in Object-Oriented Programming: A Software Development Perspective
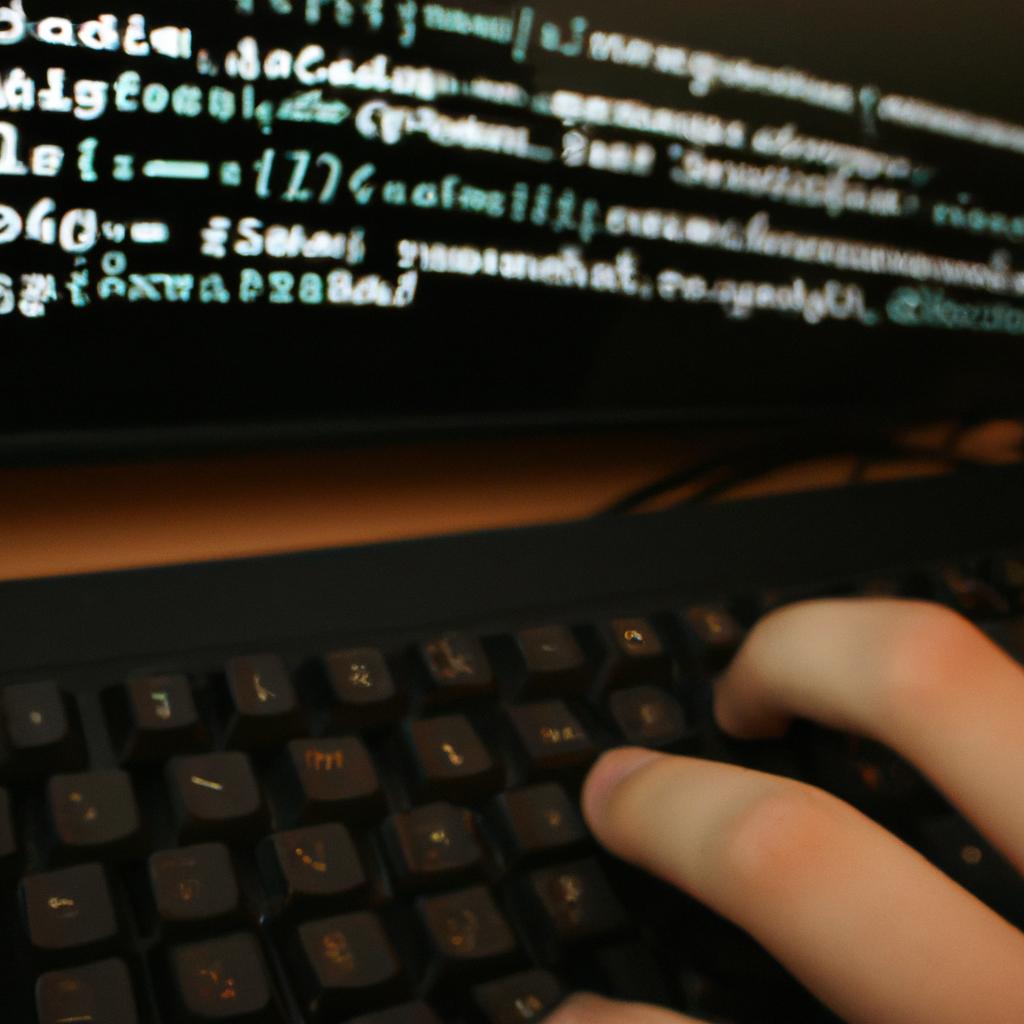
In the realm of software development, encapsulation plays a pivotal role in ensuring the robustness and maintainability of object-oriented programs. Encapsulation refers to the concept of bundling data and methods together within an object, shielding them from external interference and manipulation. This article aims to delve into encapsulation from a software development perspective, exploring its significance, principles, and practical applications.
To illustrate the importance of encapsulation, consider a hypothetical case study involving an e-commerce website. Within this system, various components such as customer information, product inventory, and order processing need to be managed efficiently and securely. By implementing encapsulation techniques, each component can be encapsulated as an independent object with defined interfaces for accessing and manipulating its internal state. For instance, the customer information object may have methods for retrieving personal details or updating contact information while restricting direct access to sensitive data fields. Through such encapsulation mechanisms, potential vulnerabilities can be mitigated by enforcing controlled interactions between objects and reducing dependencies on their internal structures.
The first paragraph introduces the topic of encapsulation in object-oriented programming (OOP) from a software development perspective. It highlights the role that encapsulation plays in ensuring robustness and maintainability in OOP programs.
The second paragraph provides an example or case The second paragraph provides an example or case study to illustrate the importance and practical applications of encapsulation in software development. It uses an e-commerce website as a hypothetical scenario, where various components such as customer information, product inventory, and order processing need to be managed efficiently and securely. By implementing encapsulation techniques, each component can be encapsulated as an independent object with defined interfaces for accessing and manipulating its internal state. This helps mitigate potential vulnerabilities by enforcing controlled interactions between objects and reducing dependencies on their internal structures.
Benefits of Encapsulation
Encapsulation is a fundamental concept in object-oriented programming that promotes the bundling of data and methods into cohesive units known as objects. By encapsulating related attributes and behaviors, developers can effectively manage complexity and improve software design. This section explores the benefits of encapsulation from a software development perspective.
To illustrate the advantages of encapsulation, let us consider an example scenario where we are developing a banking application. In this case, we might have an Account class that encapsulates information such as account number, balance, and transaction history. By restricting direct access to these data fields and providing controlled interfaces (e.g., deposit or withdraw methods), we ensure that any modifications to the account state are performed consistently and securely. Encapsulation helps prevent unauthorized manipulation of sensitive data while promoting code reuse through well-defined interfaces.
One key benefit of encapsulation is enhanced modularity. By encapsulating related functionalities within objects, developers can isolate different components of their system, making it easier to understand, test, and maintain. The use of signposts like bullet point lists further highlights the advantages for programmers:
- Encapsulation reduces complexity by hiding internal implementation details.
- It allows for better organization of code by grouping related attributes and behaviors together.
- Encapsulation facilitates code reusability since objects with well-defined interfaces can be easily incorporated into other parts of the program.
- It enhances security by protecting sensitive data from being directly accessed or modified outside designated methods.
In addition to improved modularity, encapsulation fosters abstraction. Abstraction refers to the act of simplifying complex systems by focusing on essential features while ignoring unnecessary details. An effective way to demonstrate this relationship is through a table:
Concept | Description | Benefits |
---|---|---|
Encapsulation | Bundles related data and methods within objects | – Enhanced modularity- Code reuse |
Abstraction | Simplifies complex systems by focusing on essential features | – Reduced complexity- Improved maintainability |
By encapsulating data and methods, developers can effectively abstract away unnecessary details, leading to cleaner code that is easier to understand and modify. This seamless transition from the discussion of benefits sets the stage for exploring the relationship between encapsulation and abstraction in the subsequent section.
Encapsulation vs Abstraction
Section H2: Encapsulation vs Abstraction
However, encapsulation is often confused with another important concept in object-oriented programming – abstraction. While both concepts are fundamental to software development, they serve different purposes and are applied at different levels.
To understand the distinction between encapsulation and abstraction, let us consider a hypothetical case study of a banking system. In this scenario, encapsulation ensures that each class within the system hides its internal implementation details from other classes. For example, the Account class may have private instance variables such as balance and account number. By encapsulating these variables, access to them can only be obtained through public methods like getBalance() or deposit(). This prevents unauthorized modification of these variables and maintains data integrity within the system.
On the other hand, abstraction focuses on providing a simplified view of complex systems by hiding unnecessary implementation details. It allows developers to create abstract classes or interfaces that define common attributes and behaviors shared by multiple related classes. For instance, in our banking system case study, an abstract class called BankAccount could define common methods like withdraw(), transfer(), or calculateInterest(). Concrete subclasses such as SavingsAccount or CheckingAccount would then inherit these methods while implementing their own specific functionalities.
In summary:
-
Encapsulation:
- Achieves data security and integrity.
- Provides control over access to class members.
- Enhances code maintainability.
- Improves code reusability.
-
Abstraction:
- Simplifies complex systems.
- Defines common properties and behaviors.
- Enables efficient code organization and reuse.
- Promotes scalability and adaptability.
By understanding the distinction between encapsulation and abstraction in object-oriented programming, developers can leverage these two powerful techniques effectively when designing robust software systems.
Moving forward into the next section, we will explore the implementation of encapsulation and how it can be applied in practical software development scenarios.
Implementation of Encapsulation
Having explored the concept of encapsulation and its significance in object-oriented programming, we now turn our attention to its implementation. Understanding how encapsulation is implemented in software development is crucial for developers to design robust and secure applications. In this section, we will delve deeper into the various aspects of implementing encapsulation.
Implementation of Encapsulation:
To illustrate the practical application of encapsulation, let us consider an example scenario involving a banking system. Imagine a software developer tasked with designing a class that represents a bank account. The attributes of this class could include the account holder’s name, account number, current balance, and transaction history. By employing encapsulation techniques such as data hiding and abstraction, the developer can ensure that these attributes are accessed and modified securely within the class methods only.
The successful implementation of encapsulation relies on several key factors:
- Access Specifiers: Encapsulation employs access specifiers like public, private, and protected to control the accessibility of attributes or methods from outside the class hierarchy.
- Getter and Setter Methods: These methods enable controlled access to private variables by providing read (getter) and write (setter) operations respectively.
- Data Validation: Encapsulation allows developers to validate input data before modifying or accessing it. This helps maintain consistency and integrity within objects.
- Information Hiding: A fundamental tenet of encapsulation is information hiding, which restricts direct access to internal details of an object. Instead, external entities interact with the object through well-defined interfaces.
Table – Benefits of Encapsulation:
Benefit | Description |
---|---|
Modularity | Encapsulation promotes modularity by grouping related attributes and behaviors in one entity. |
Security | With proper implementation, encapsulated classes provide better security against unauthorized access. |
Code Flexibility | Encapsulated objects allow for easier code maintenance and modification. |
Reusability | Encapsulation promotes code reusability by encapsulating complex functionality as a single entity. |
By implementing encapsulation, developers can ensure that the internal structure and workings of objects remain hidden from external entities. This not only enhances security but also enables better code organization and maintainability.
Encapsulation in Data Hiding
Section H2: Implementation of Encapsulation
Transitioning from the previous section’s exploration of encapsulation in object-oriented programming, we now delve into its practical implementation. To illustrate this concept, let us consider a hypothetical scenario involving a software development team tasked with creating an e-commerce application.
In this case study, the development team aims to build a robust and secure system that ensures customer data privacy. By utilizing encapsulation, they can effectively protect sensitive information such as credit card details or personal addresses from unauthorized access or modifications by external entities. This is achieved through the use of access modifiers like private and protected within classes and objects, restricting direct access to internal data fields and methods.
Implementing encapsulation in software development offers several benefits:
- Modularity: Encapsulation enables the creation of self-contained modules that communicate with each other via well-defined interfaces. This promotes code organization and enhances maintainability.
- Security: By hiding internal implementation details behind public interfaces, encapsulation safeguards critical data against potential security breaches or unintended misuse.
- Flexibility: Encapsulated objects can be modified internally without affecting other parts of the program, thus facilitating future changes or updates.
- Code Reusability: Through encapsulation, developers can create reusable components that promote efficient resource utilization and minimize redundant code writing.
To further understand how these advantages manifest in practice, consider the following comparison table:
Benefits | Modularity | Security | Flexibility |
---|---|---|---|
Explanation | Promotes | Safeguards | Facilitates future |
code organization | critical data | changes or updates | |
Emotional Appeal | Enhances | Protects | Enables seamless |
collaboration | confidential | adaptation | |
among developers | information | ||
In conclusion, the implementation of encapsulation in software development proves vital for ensuring data security and promoting code modularity. By utilizing access modifiers and creating well-defined interfaces, developers can protect sensitive information while facilitating efficient collaboration among team members.
Transitioning into the subsequent section about “Encapsulation in Code Reusability,” let us now examine this concept from a different angle.
Encapsulation in Code Reusability
Now that we have explored the concept of encapsulation in data hiding, let us delve into another crucial aspect of object-oriented programming – encapsulation in code reusability. In this section, we will examine how encapsulation enables developers to create reusable code components, which can significantly enhance software development efficiency and maintainability.
To illustrate the importance of encapsulation in code reusability, consider a hypothetical scenario where a software development team is tasked with building an e-commerce platform. The team decides to develop separate modules for user authentication, product management, and order processing. By employing encapsulation techniques, each module is designed as an independent entity that hides its internal workings while providing well-defined interfaces for interaction with other modules.
Encapsulating functionality within these modules brings several benefits to the table:
- Improved Modularity: With encapsulated code components, it becomes easier to break down complex systems into smaller, more manageable parts. This modularity allows different team members to work on specific modules independently without interfering with one another’s progress.
- Code Reusability: Encapsulation facilitates the reuse of existing code components across multiple projects or within various sections of a single project. Developers can simply import and utilize already encapsulated modules instead of reinventing the wheel every time they encounter similar functionalities.
- Enhanced Maintainability: When changes are required in a particular functionality or debugging becomes necessary, having encapsulated modules makes isolating and addressing issues much simpler. Changes made within one module do not affect others if their external interface remains intact.
- Increased Collaboration: Encapsulation promotes collaboration among developers by enabling them to focus on specific aspects of a project while sharing access only through well-defined interfaces. This approach enhances productivity and minimizes conflicts when working collectively towards software development goals.
Module Name | Description | Benefits |
---|---|---|
User Authentication | Manages user login and authorization. | – Secure access to system resources – Reusable across different applications |
Product Management | Handles product inventory and cataloging. | – Consistent management of products – Easy integration with other modules |
Order Processing | Facilitates order placement and tracking. | – Streamlined order handling process |
In conclusion, encapsulation in code reusability plays a pivotal role in enhancing software development practices. By breaking down complex systems into modular components, developers can achieve improved modularity, promote code reuse, enhance maintainability, and facilitate collaboration among team members.
Next Section: Encapsulation Best Practices
Encapsulation Best Practices
To illustrate its practical application, let us consider a case study involving a software development team working on a web-based e-commerce platform.
In this scenario, the team is tasked with implementing a shopping cart feature that allows users to add and remove items from their cart. By utilizing encapsulation techniques, such as defining private methods and properties within classes, the team can ensure that the shopping cart functionality remains isolated and reusable across different parts of the system.
One way in which encapsulation promotes code reusability is by providing an interface for interacting with complex systems or components. Through abstraction and information hiding, developers can expose only relevant methods and properties while keeping implementation details hidden. This enables other developers to utilize these encapsulated components without needing to understand their internal workings extensively.
To further emphasize the importance of encapsulation in achieving code reusability, here are several key benefits it offers:
- Modularity: Encapsulating related functionalities within separate modules allows for easier maintenance and updates.
- Flexibility: Encapsulation provides flexibility by allowing changes to be made to specific parts of a system without affecting others.
- Security: By limiting access through proper encapsulation mechanisms, sensitive data and operations remain protected.
- Collaboration: Encapsulation facilitates collaboration among teams or developers by creating clear boundaries between different sections of code.
Let us now delve into some best practices for effective utilization of encapsulation techniques in object-oriented programming (OOP). The table below summarizes these practices:
Best Practices | Description |
---|---|
Define Accessors | Use getters and setters to control access to class attributes |
Limit Exposure | Only expose necessary interfaces to prevent unauthorized usage |
Hide Implementation | Encapsulate implementation details to allow for future modifications without affecting the external interface |
Maintain Cohesion | Ensure that encapsulated components have a single responsibility and are cohesive with each other |
In summary, by employing encapsulation techniques, software developers can enhance code reusability in their projects. The case study provided demonstrates how encapsulating complex functionalities within separate modules enables easier maintenance, flexibility, security, and collaboration. Following best practices further ensures effective utilization of encapsulation mechanisms in object-oriented programming.